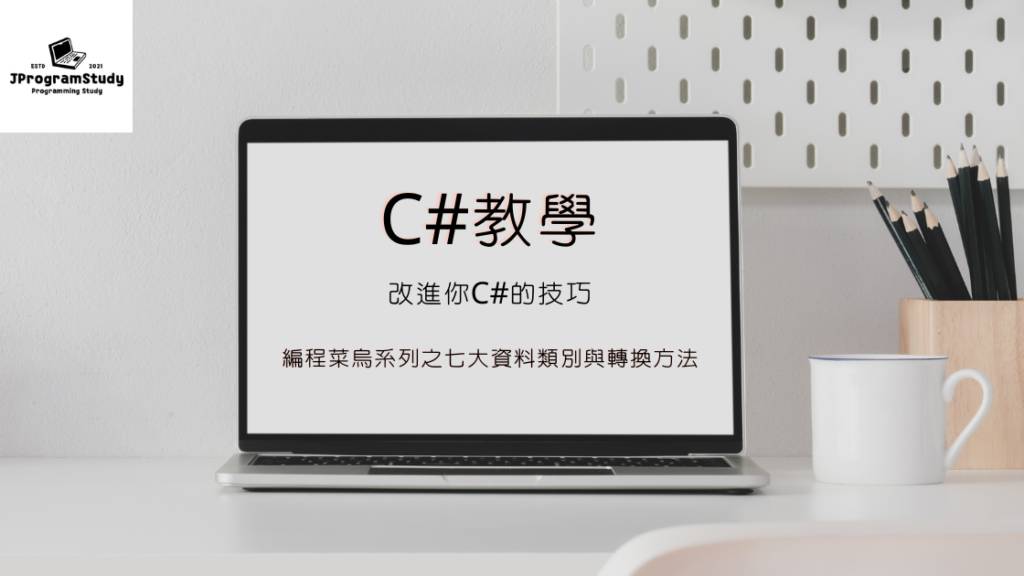
資料類別一覽表
資料類別 | 大小 | 數值範圍 | 例子 |
int | 4 bytes | 整數: -2,147,483,648 至 2,147,483,647 | int value = 123456; |
long | 8 bytes | 整數: -9,223,372,036,854,775,808至9,223,372,036,854,775,807 | long value = 123456789012; |
float | 4 bytes | 小數: 最多6 至 7 個小數 | float value = 123.456f; |
double | 8 bytes | 小數: 最多15 個小數 | double value = 123.4567890; |
bool | 1 bit | 對錯: 只有 true 及 false 兩個值 | bool value = true; |
char | 2 bytes | 字元 / 字母 | char value = ‘H’; |
string | 每個字元2 bytes | 字串 | string value = “Good Morning!”; |
數值文字轉換方法
資料類別 | Convert | 例子 |
int | Convert.ToInt32(“數值”) | int value = Convert.ToInt32(“21”); |
long | Convert.ToInt64(“數值”) | long value = Convert.ToInt64 (“64.1”); |
float | Convert.ToFloat(“數值”) | float value = Convert.ToFloat (“64.1”); |
double | Convert.ToDouble(“數值”) | double value = Convert.ToDouble (“64.1”); |
bool | Convert.ToBoolean(“數值”) | bool value = Convert.ToBoolean (“1”); |
char | Convert.ToChar(“數值”) | char value = Convert.ToChar(“13”); |
string | “值”.ToString() | string value = “64.1”.ToString(); |
資料類別 | TryParse | Parse |
int | Int32.TryParse(“string 值”, out “int 數值”) | int value = Int32.Parse(“string 值”) |
long | Int64.TryParse(“string值”, out “long 數值”) | long value = Int64.Parse(“string值”) |
float | float.TryParse(“string值”, out “float 數值”) | float value = float.Parse(“string值”) |
double | double.TryParse(“string值”, out “double 數值”) | double value = double.Parse(“string值”) |
bool | bool.TryParse(“string值”, out “bool 數值”) | bool value = bool.Parse(“string值”) |
char | char.TryParse(“string值”, out “char 數值”) | char value = char.Parse(“string值”) |
Widening Casting (自動) – 把較小bytes位的數值轉至較大bytes位的數值 | byte > short > char > int > long > float > double |
Narrowing Casting (人手) – 把較大bytes位的數值轉至較小bytes位的數值 | double > float > long > int > char > short > byte |
其他 – 程序實作
Const Keyword 常量字段和局部變量不是變量,不能修改。 e.g. public const double GravitationalConstant = 6.673e-11; | Static Keyword static關鍵字屬於類而不是類的實例。 你能通過類直接CALL, 而不能, 也不用類的Instance Call. Public class test { public static int x = 100; } Console.WriteLine(Test.x) // OK Console.WriteLine(new Test().x) // Fail |
Global, Local Scope | |
Public class car { int engineOil; Public Run(int speed) { Int expense = engineOil * speed; } } | Global scope variable speed 能在Class 內調用, run方法內外調用都能 Local scope variable engineOil, speed 不能在run方法外調用 |