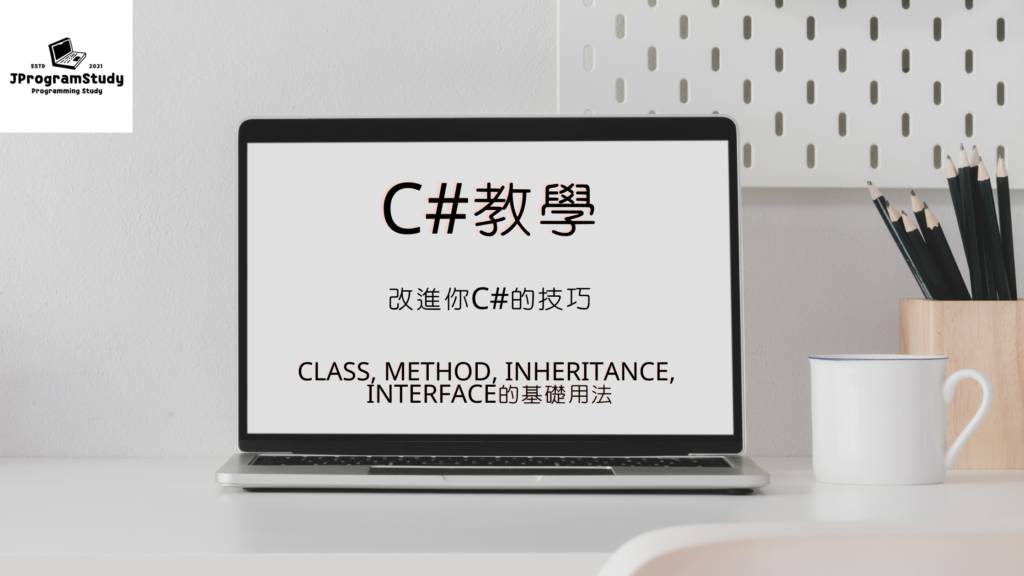
C#類別 – 本篇會介紹 C# Class, C# Method, C# Inheritance, C# Interface的基礎用法. Let’s Start!
句型 | 例子: |
interface (父類別名稱){ //父類別內容 } | class Person{ public string name; public string surname; public Person(string name, string surname){ this.name = name; this.surname = surname; } public string GetFullName(){ return this.name + ” ” + this.surname; } } |
句型 | 例子: |
class (類別名稱) : (抽象類別名稱){ //類別內容 //可引用父類別內容 //在例子中: string GetFullName } | class Student : Person { public int chiGrade; public int engGrade; public int mathsGrade; public Student(string name, string surname, int chiGrade , int engGrade, int mathsGrade) : base(name, surname) { this.chiGrade = chiGrade; this.engGrade = engGrade; this.mathsGrade = mathsGrade; } public bool isChiPassed() { if (chiGrade < 50) { return false; } return true; } //Omit …. } |
class (類別名稱) : (抽象類別名稱){ //類別內容 //可引用父類別內容 //在例子中: string GetFullName } | class Teacher : Person{ public int teachingGrade; public string schoolName; public Teacher(string name, string surname, string schoolName, int teachingGrade) : base(name, surname) { this.schoolName = schoolName; this.teachingGrade = teachingGrade; } public bool isTeachingPassed() { if (chiGrade < 50) { return false; } return true; } } |
句型 | 例子: |
interface (介面名稱){ //介面內容 } | interface ICheckedPassed{ bool isPassed(); } |
句型 | 例子: |
class (類別名稱) : (介面名稱){ //類別內容 //必須展開介面內容 //在例子中: bool isPassed() } | class Student : Person, ICheckedPassed{ public int chiGrade; public int engGrade; public int mathsGrade; //Omit …. public bool isPassed(){ return isChiPassed() && isEngPassed() && isMathsPassed(); } } |
句型 | 例子: |
class (類別名稱) : (介面名稱){ //類別內容 //必須展開介面內容 //在例子中: bool isPassed() } | class Teacher : Person{ public int teachingGrade; public string schoolName; //Omit …. public bool isPassed(){ return isTeachingPassed(); } } |
相關頁面:
C#繼承 – Inheritance 繼承 的基本用法 – 加入 for loop, if, Array, Collection的例子
C#介面 – Interface 介面 的基本用法 – 加入 for loop, if, Array, Collection的例子
參考資料: https://docs.microsoft.com/en-us/dotnet/csharp/fundamentals/object-oriented/inheritance