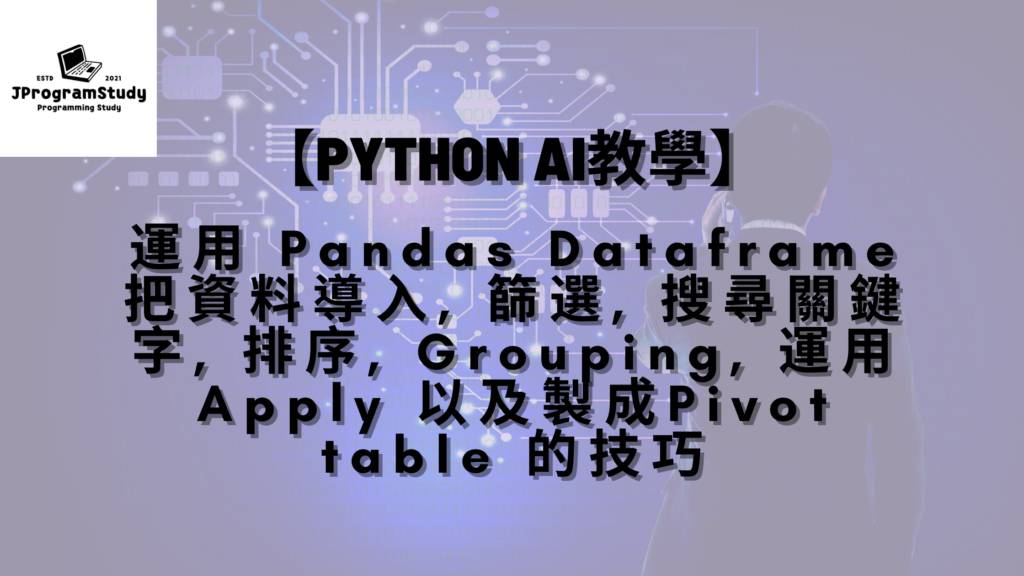
Pandas Dataframe – 在本文中,我將向你展示利用Python AI Pandas 資料庫的Dataframe, 來導入, 篩選, 排序, Grouping資料, 搜尋關鍵字, 以及製成Pivot table 的技巧。
安裝資料庫 | pip install pandas |
導入資料庫 | import pandas as pd |
把資料導入dataframe | |
設定Dictionary 資料 | students = { “name”: [“Johnson”, “Windy”, “Dickson”, “Jenny”], “age”: [14, 16, 14, 15], “email”: [“js@ptschool.com”, “wffy@gmail.com”, “dickson2004@gmail.com”, “hello12344321@hotmail.com”], “chinese”: [90, 89, 33, 78], “english”: [88, 56, 65, 67],} |
把Dictionary 資料導入dataframe | student_df = pd.DataFrame(students) |
資料篩選 | |
以variable進行資料篩選 | marks_over_80 = (df. english >= 80) & (df. chinese >= 80)print(df[ marks_over_80 ]) |
以括號進行資料篩選 | print(df[df[“english”] > 80 & df[“chinese”] > 80]) |
以query進行資料篩選 | english = 80chinese = 80df.query(“english > @english & chinese > @chinese) |
以日期進行資料篩選(5週) | df . last_login .first(‘5W’) |
以Regex進行資料篩選 | df . last_login .filter(regex=”2021-12.*”, axis=0) |
Apply運用 | |
以apply讓每一列也自動進行method | def generate_status(row):return f”{row[‘name’]}現年{row[‘age’]}歲”df[‘status’] = df.apply(generate_status, axis=1) |
以apply讓每一列也自動進行if | df[‘engPass’] = df.english.apply(lambda x: ‘合格’ if x >= 60 else ‘不合格’) |
在字串中搜尋查詢關鍵字 | |
Option 1 | print(df[df[“name”].isin([“Johnson”])]) |
Option 2 | print(df[df.Name.str.contains(” John\.”)]) |
資料排序 | |
依索引值排序 | new_df = df.sort_index(ascending=True) |
依欄位內容排序 | new_df = df.sort_values([“chinese”], ascending=True) |
Grouping | |
產生欄位的樣本數: | df.groupby([“age”, ‘chinese’]).size().unstack() |
用 agg 做匯總 | df.groupby([“age”, ‘chinese’]).Age.agg([‘min’, ‘max’, ‘count’]) |
用 unstack 產生跟 pivot_table 相同的結果 | df.groupby([“age”, ‘chinese’]).Age.agg([‘min’, ‘max’, ‘count’]).unstack() |
Group欄位後產生其他欄位的中位數 | |
Option 1 | df[‘Avg_age’] = df.groupby(“age”).chinese.transform(“mean”) |
Option 2 | df.groupby(“Pclass”).Age.mean() |
Pivot table | |
產生 pivot_table 來匯總各組數據 | df.pivot_table(index=’Sex’, columns=’Pclass’, values=’Age’, aggfunc=[‘min’, ‘max’, ‘count’]) |