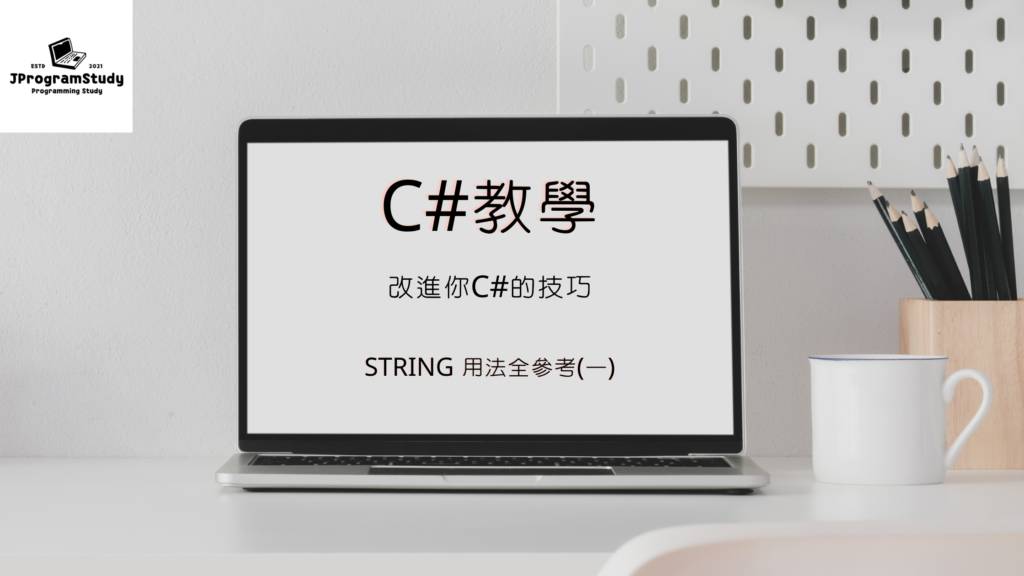
C# 字串 – 以下我會介紹:
- C# 字串插入 (C# Insert)
- C# 字串移除 (C# Remove)
- C# 字串替換 (C# Replace)
- 移除空白 (C# Trim)
- C# 大小寫 (C# ToUpper, C# ToLower)
- C# 字串合併, C# 字串分割 (C# Split).
Let’s start for our study!
Title 1: C# 字串插入, c# 字串移除, C# 字串替換 - C# Insert, C# Remove, C# Replace
這一段,我將向你展示c#程式設計的3個技巧, 就是把string字串進行插入, 移除, 取代的方法。
C# Insert - string Insert (c# 字串插入):
以下為String.Insert(Int32, String) , 在字串中插入文字的方法:
String.Insert("從第幾個字開始加入", "加入的字串"):
```
string str = "I can fly";
Console.WriteLine(str.Insert(2,"believe I "));
//結果: I believe I can fly
```
C# Remove - string Remove (c# 字串移除):
以下為String.Remove(Int,Int) , 在字串中移除文字的方法:
String.Remove("從第幾個開始移除", 移除多少字元);
```
string str = "I believe I can fly";
Console.WriteLine(str.Remove(2, 10));
//結果: I can fly
```
C# Replace - string Replace (C# 字串替換):
以下為String.Replace() , 取代字串的方法:
String.Replace(String)
String.Replace(取代的字串目標,新的字串)
```
string str = "I am sure I can fly";
string strResult = str.Replace("am sure", "believe");
Console.WriteLine(strResult);
//結果: I believe I can fly
```
以下為Regex.Replace() , C# 字串取代 (忽略大小寫)的方法:
Regex.Replace(String, String , String, RegexOptions.IgnoreCase);
Regex.Replace(輸入字串, 取代的字串目標,新的字串, RegexOptions.IgnoreCase);
```
string str = "I am sure I can fly";
string strResult = Regex.Replace(str, "AM SURE", "believe", RegexOptions.IgnoreCase);
Console.WriteLine(strResult);
//結果: I believe I can fly
```
Title 2: C# 移除空白, 補上空白string字串 - C# Trim, C# TrimStart, C# TrimEnd, C# PadLeft, C# PadRight
這一段,我將向你展示c#程式設計的2個技巧, 就是把string字串移除空白或補上空白的方法。
C# Trim - string Trim - 移除空白
以下為String.Trim() , 移除字串兩邊的空白的方法:
```
string str = " $10000 ";
string strResult = str.Trim();
Console.WriteLine(strResult);
//結果: "$10000"
```
C# TrimStart
以下為String.TrimStart() , 移除字串開頭的空白的方法:
```
string str = " $10000 ";
string strResult = str.TrimStart();
Console.WriteLine(strResult);
//結果: "$10000 "
```
C# TrimEnd
以下為String.TrimEnd () , 移除字串結尾的空白的方法:
```
string str = " $10000 ";
string strResult = str.TrimEnd();
Console.WriteLine(strResult);
//結果: " $10000"
```
C# PadLeft
以下為String.PadLeft(Int32), 為字串左邊補上空白的方法:
String.PadLeft(字串總數包括補上的空白格)
```
string str = "$10000";
string strResult = str.PadLeft(10);
Console.WriteLine(strResult);
//結果: " $10000"
```
以下為String.PadLeft(Int32, char), 為字串左邊補上字元的方法:
String.PadLeft(字串總數包括補上的空白格)
```
string str = "$10000";
string strResult = str.PadLeft(10, '-');
Console.WriteLine(strResult);
//結果: "----$10000"
```
C# PadRight
以下為String.PadRight(Int32), 為字串右邊補上空白的方法:
String.PadRight(字串總數包括補上的空白格)
```
string str = "$10000";
string strResult = str.PadRight(10);
Console.WriteLine(strResult);
//結果: "$10000 "
```
以下為String.PadRight(Int32, char), 為字串右邊補上字元的方法:
String.PadRight(字串總數包括補上的空白格)
```
string str = "$10000";
string strResult = str. PadRight(10, '-');
Console.WriteLine(strResult);
//結果: "$10000----"
```
Title 3: 轉換string字串成大小寫 - C# ToUpper, C# ToLower
在本段中,我將向你展示c#程式設計的2個技巧, 就是把string字串轉換成大寫或小寫方法。
C# ToLower
以下為String.ToLower(), 把string字串轉換成大寫的方法:
```
string str = "I believe I can fly";
string strResult = str. ToLower();
Console.WriteLine(strResult);
//結果: i believe i can fly
```
C# ToUpper
以下為String.ToUpper(), 把string字串轉換成小寫的方法:
```
string str = "I believe I can fly";
string strResult = str.ToUpper();
Console.WriteLine(strResult);
//結果: I BELIEVE I CAN FLY
```
Title 4: string C#字串合併, C#字串分割 - C# Split
在本段中,我將向你展示c#程式設計的2個技巧, 就是把string字串進行成合併或分割。
C# Split - 字串合併
以下為String.Join(String, String[]), 將字串維度合併的方法:
String.Split(中間字串, 合併字串維度)
```
string[] strs = new string[]{"I believe", "I can fly"};
string strResult = String.Join(" ", strs);
Console.WriteLine(strResult);
//結果: I BELIEVE I CAN FLY
```
以下為String.Split(char), 將字串用字元(char, 非string字串)分割:
String.Split('用來分割的字元')
```
string str = "I believe !I can fly";
string[] strResult = str.Split('!');
foreach (var item in strResult)
Console.WriteLine(item);
//結果:
//I believe
//I Can fly
```
Regex.Split - 將字串分割
以下為Regex.Split(String, String), 將字串用string字串分割:
Regex.Split(分割字串對象,"用來分割的字串")
```
string str = "I believe that I can fly";
string[] strResult = Regex.Split(str, " that ");
foreach (var item in strResult)
Console.WriteLine(item);
//結果:
//I believe
//I Can fly
```
Title 5: 在string字串前加「@」或「$」, 了解兩者的意思及用法。
以下,我將向你展示c#程式設計的2個技巧, 就是在string字串前加「@」或「$」, 這應該可以令編程更容易。
1. 在string字串前加「@」的意思及用法
2. 在string字串前加「$」的意思及用法
在string字串前加「@」的意思及用法
若果不加「@」的話, 如果string內包含Blackslash 「\」的話 , 就必須用Escape Character「\\」表示。
但若在string字串前加「@」包含Blackslash 「\」, 不用Escape Character「\\」, 如下例:
```
string str = "c:\\temp"
//等如
string str = @"c:\temp"
```
其他的例子還有:
Description Escape character
Single quote (') \'
Double quote (") \"
Backslash (\) \\
在string字串前加「$」的意思及用法
從C#6.0開如, C# 以「$」簡化了string.format 的用法, 以下為一個例子:
用string.format的話, 會比較麻煩:
```
var lastName = "間";
var firstName = "碟";
var codeNo = "007";
var result = string.format("我姓{0}, 名{1}, 編號{2}", lastName, firstName, codeNo);
Console.WriteLine(result);
```
結果如下:
```
我姓間, 名碟, 編號007
```
用「$」的話, 就簡化了很多:
```
var lastName = "間";
var firstName = "碟";
var codeNo = "007";
var result = $"我姓{ lastName }, 名{firstName}, 編號{codeNo}";
Console.WriteLine(result);
```
結果如下:
```
我姓間, 名碟, 編號007
```
下一編:
其他相關:
C# 字串String功能速查表 – 尋找, 擷取, 對比, 組合
C# 字串String功能速查表 – 插入, 移除, 取代, 移除空白, 補上空白, 轉換成大小寫, 合併分割
參考資料:
https://docs.microsoft.com/en-us/dotnet/api/system.string?view=net-6.0