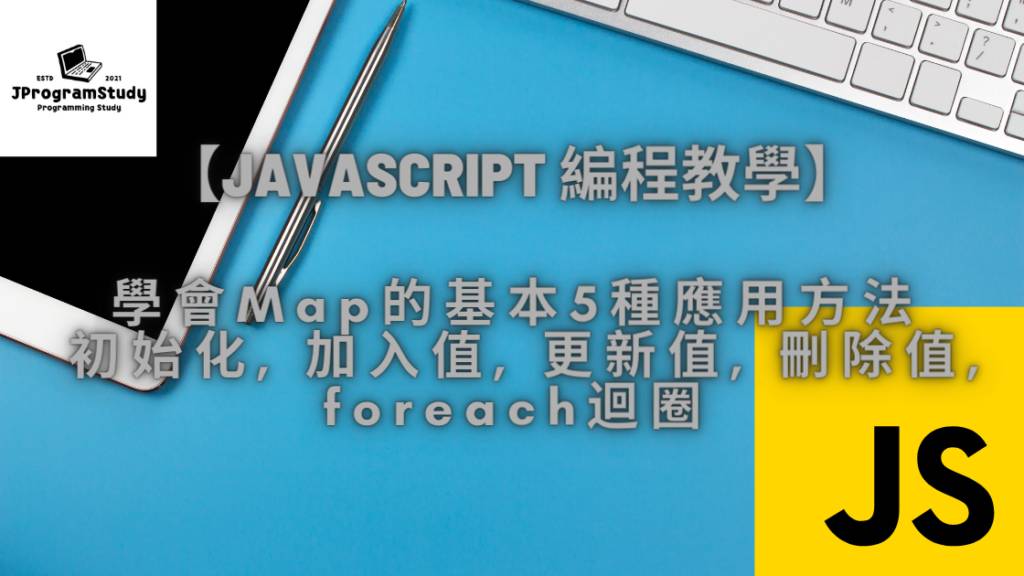
JS Map – 本篇會介紹Map的5種基本應用方法 – Map初始化, Map加入值, Map更新值, Map刪除值, Map foreach迴圈. Let’s Start!
方法 | 例子: |
初始化 Map Init | const names = new Map([]); console.log(names); // Map { } |
初始化 Map Init | const names = new Map([ [“Tim”, “Clerk”], [“John”, “Manager”], [“Mary”, “Boss”]]); console.log(names); // Map { ‘Tim’ => ‘Clerk’, ‘John’ => ‘Manager’, // ‘Mary’ => ‘Boss’ } |
加入值 Map set | const names = new Map([]); names.set(“Tim”, “Clerk”); names.set (“John”, “Manager”); names.set (“Mary”, “Boss”); console.log(names); // Map { ‘Tim’ => ‘Clerk’, ‘John’ => ‘Manager’, // ‘Mary’ => ‘Boss’ } |
獲取值 Map get | const names = new Map([]); names.set(“Tim”, “Clerk”); names.set (“John”, “Manager”); names.set (“Mary”, “Boss”); console.log(names.get(“Tim”)); // Clerk |
更新值 Map has Map set | const names = new Map([]); names.set(“Tim”, “Clerk”); names.set(“John”, “Manager”); names.set(“Mary”, “Boss”); if (names.has(“Tim”)){ names.set(“Tim”, “Cooker”); } console.log(names.get(“Tim”)); //Cooker |
刪除值 Map delete | const names = new Map([]); names.set(“Tim”, “Clerk”); names.set(“John”, “Manager”); names.set(“Mary”, “Boss”); names.delete(“Tim”); console.log(names.get(“Tim”)); //undeifined |
for迴圈 Map For | const names = new Map([]); names.set(“Tim”, “Clerk”); names.set (“John”, “Manager”); names.set (“Mary”, “Boss”); for (const x of names.entries()) { console.log(x); } //[ ‘Tim’, ‘Clerk’ ] //[ ‘John’, ‘Manager’ ] //[ ‘Mary’, ‘Boss’ ] |
(等如) | |
foreach迴圈 Map ForEach | const names = new Map([]); names.set(“Tim”, “Clerk”); names.set (“John”, “Manager”); names.set (“Mary”, “Boss”); names.forEach (function(value, key) { console.log([key, value]); }) //[ ‘Tim’, ‘Clerk’ ] //[ ‘John’, ‘Manager’ ] //[ ‘Mary’, ‘Boss’ ] |
相關頁面:
JS 陣列 – 學會Array陣列基本8種應用方法 – 初始化, 加入值, 插入值, 更新值, 刪除值, 抽取值, 排序, foreach迴圈
JS Set – 學會Set的基本4種應用方法 – 初始化, 加入值, 刪除值, foreach迴圈
資料參考: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Map