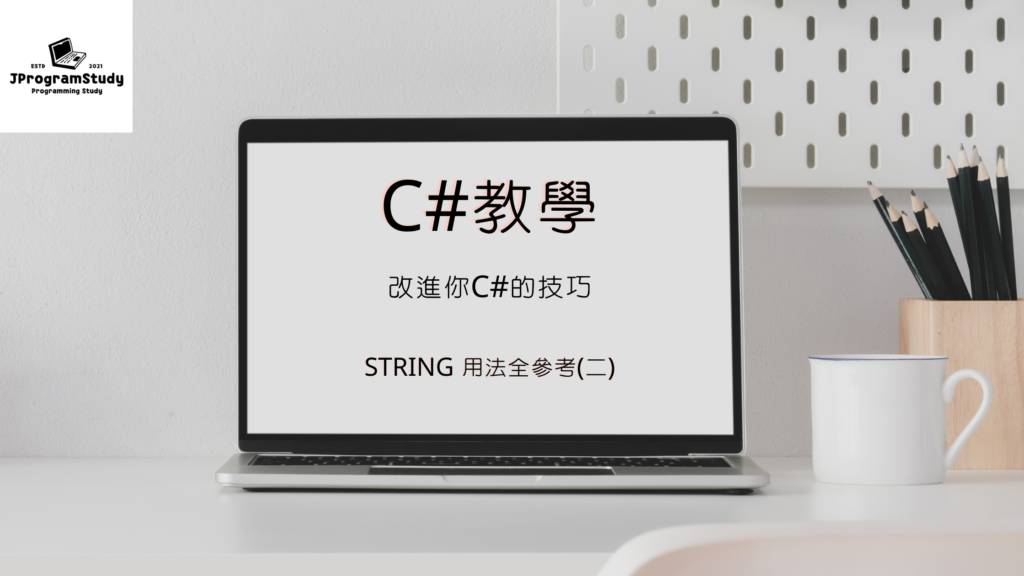
C#字串 string – 以下我會介紹幾種string 的 Method:
- C# 尋找字串(C# Contains, C# StartsWith, C# EndsWith, C# IndexOf, C# LastIndexOf, C# IsMatch)
- C# 擷取字串 (C# Substring)
- C# 字串合併, C# 字串分割 (C# Join, C# Split)
- C# 組合字串 (C# Concat, C# Join)
- C# 對比字串 (C# Equals, C# CompareTo, C# IsNullOrEmpty, C# IsNullOrWhiteSpace)
Let’s go for our study!
C# 擷取字串 — C# Substring
以下為String.Substring(Int32, Int32) , 撷取字串的方法:
String.Substring(由第幾個字元開始撷取, 要撷取多少個);
“`
string str = “I believe I can fly”;
Console.WriteLine(str.Substring(2, 7));
//結果: believe
“`
C# 字串合併, C# 字串分割 — C# Join, C# Split
以下為String.Join(String, String[]), 將字串維度合併的方法:
String.Split(中間字串, 合併字串維度)
“`
string[] strs = new string[]{“I believe”, “I can fly”};
string strResult = String.Join(” “, strs);
Console.WriteLine(strResult);
//結果: I BELIEVE I CAN FLY
“`
以下為String.Split(char), 將字串用字元(char, 非string字串)分割:
String.Split(‘用來分割的字元’)
“`
string str = “I believe !I can fly”;
string[] strResult = str.Split(‘!’);
foreach (var item in strResult)
Console.WriteLine(item);
//結果:
//I believe
//I Can fly
字串分割 – Regex.Split
以下為Regex.Split(String, String), 將字串用string字串分割:
Regex.Split(分割字串對象,”用來分割的字串”)
“`
string str = “I believe that I can fly”;
string[] strResult = Regex.Split(str, ” that “);
foreach (var item in strResult)
Console.WriteLine(item);
//結果:
//I believe
//I Can fly
“`
C# 組合字串 — C# Concat, C# Join
以下為String.Concat(String, String) , 字串組合 的方法:
String.Concat(“字串1”, “字串2”);
“`
string str1 = “I believe I can fly. “;
string str2 = “I believe I can talk to sky. “;
Console.WriteLine(String.Concat(str1, str2));
//結果: “I believe I can fly. I believe I can talk to sky.”
“`
以下為String.Join(String, String[]) , 字串數組組合 的方法:
String.Join(“接合字串”, “字串數組”);
“`
string[] strs = new string[] { “I”, “believe”, “I”, “can”, “fly.” };
Console.WriteLine(String.Join(“ “, strs));
//結果: “I believe I can fly.
“`
C# 對比字串 — C# Equals, C# CompareTo, C# IsNullOrEmpty, C# IsNullOrWhiteSpace
字串對比 (包含大小寫對比)
以下為String.Equals(String), 字串對比 (包含大小寫對比)的方法:
String.Equals(“要對比的字串”);
“`
string str1 = “I believe I can fly”;
string str2 = “i believe i can fly”;
Console.WriteLine(str1.Equals(str2));
“`
以下為String.Equals(String, StringComparison) , 字串對比 (不包含大小寫對比)的方法:
String.Equals(“要對比的字串”, StringComparison.CurrentCultureIgnoreCase);
“`
string str1 = “I believe I can fly”;
string str2 = “i believe i can fly”;
Console.WriteLine(str1.Equals(str2, StringComparison.CurrentCultureIgnoreCase));
“`
以下為String.CompareTo(String), 字串對比的方法:
String.CompareTo(“要對比的字串”):
*回傳值: 0= 相同; -1 = 不相同
“`
string str1 = “I believe I can fly”;
string str2 = “I sure I can fly”;
Console.WriteLine(str1.CompareTo(str2));
“`
以下為String.IsNullOrEmpty( String), 查詢字串是不為空值(null)或空字串(“”)的方法:
String.IsNullOrEmpty(“要對比的字串”):
“`
string str = “ “;
Console.WriteLine(String.IsNullOrEmpty(str));
“`
以下為String.IsNullOrWhiteSpace(String) , 查詢字串中是不為空值(null)或空字串(“”)或空格(“ “)的方法:
String.IsNullOrWhiteSpace(“要對比的字串”);
“`
string str = “ “;
Console.WriteLine(String.IsNullOrWhiteSpace(str));
“`
C# 尋找字串 — C# Contains, C# StartsWith, C# EndsWith, C# IndexOf, C# LastIndexOf, C# IsMatch
尋找string字串
以下為String.Contains( String), 是否存在要搜查的關键句於字串的方法:
String.Contains(“要搜查的關键句”):
“`
string str = “I believe I can fly”;
Console.WriteLine(str.Contains(“believe”));
“`
以下為String.StartsWith(String), 搜查的關键句是否存在於字串的開端的方法:
String.StartsWith(“要搜查的關键句”):
“`
string str = “I believe I can fly”;
Console.WriteLine(str.StartsWith(“I believe”));
“`
以下為String.EndsWith(String), 搜查的關键句是否存在於字串的尾端的方法:
String.EndsWith(“要搜查的關键句”):
“`
string str = “I believe I can fly”;
Console.WriteLine(str.EndsWith(“believe”));
“`
以下為String.IndexOf(String) , 搜查的關键句存在於字串中的第幾個字元(由前到後)的方法:
String.IndexOf(“要搜查的關键句”):
“`
string str = “I believe I can fly”;
Console.WriteLine(str.IndexOf(“I”));
“`
以下為String.LastIndexOf(String) , 搜查的關键句是存在於字串中的第幾個字元(由後到前)的方法:
String.LastIndexOf(“要搜查的關键句”):
“`
string str = “I believe I can fly”;
Console.WriteLine(str.LastIndexOf(“I”));
//結果: 10
“`
以下為Regex.IsMatch(String, String) , 搜查的關键句是存在於字串中的第幾個字元(由後到前)的方法:
Regex.IsMatch(“搜查的對象字串”, “要搜查的關键句”);
“`
string str = “I believe I can fly”;
Console.WriteLine(Regex.IsMatch(“believe”));
“`
上一編:
其他相關:
C# 字串String功能速查表 – 尋找, 擷取, 對比, 組合
C# 字串功能速查表 – 插入, 移除, 取代, 移除空白, 補上空白, 轉換成大小寫, 合併分割
參考資料:
https://docs.microsoft.com/en-us/dotnet/api/system.string?view=net-6.0