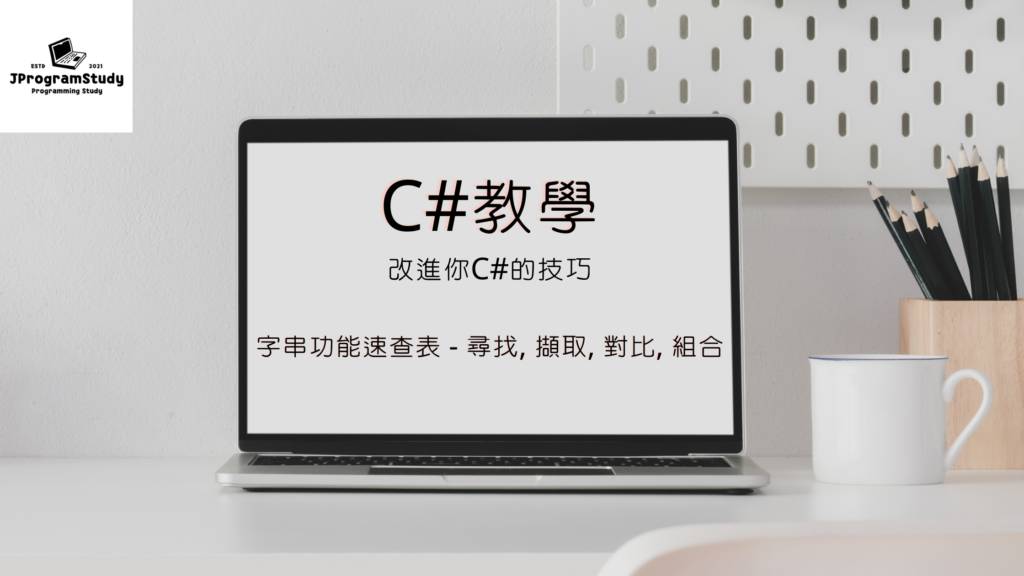
C# 字串 – 在本文中,我將向你展示在c#程式設計中, 字串普遍都會使用的14方法, 當中的功能為在字串上進行尋找, 擷取, 對比, 組合
方法 | 例子: |
搜查的關键字是否存在於字串中: String.Contains(String); String.Contains(“要搜查的關键句”); | string str = “I believe I can fly”; Console.WriteLine(str.Contains(“believe”)); //結果: True |
搜查的關键字是否存在於字串的開端: String.StartsWith(String); String.StartsWith(“要搜查的關键句”); | string str = “I believe I can fly”; Console.WriteLine(str.StartsWith(“I believe”)); //結果: True |
搜查的關键字是否存在於字串的尾端: String.EndsWith(String); String.EndsWith(“要搜查的關键句”); | string str = “I believe I can fly”; Console.WriteLine(str.EndsWith(“believe”)); //結果: False |
搜查的關键字位於字串中的第幾個字元(由前到後): String.IndexOf(String); String.IndexOf(“要搜查的關键句”); *若回傳值”-1″, 即是找不到. | string str = “I believe I can fly”; Console.WriteLine(str.IndexOf(“I”)); //結果: 0 |
搜查的關键字位於字串中的第幾個字元 (由後到前): String.LastIndexOf(String); String.LastIndexOf(“要搜查的關键句”); *若回傳值”-1″, 即是找不到. | string str = “I believe I can fly”; Console.WriteLine(str.LastIndexOf(“I”)); //結果: 10 |
搜查的關键字位於字串中的第幾個字元 (由後到前): Regex.IsMatch(String, String); Regex.IsMatch(“搜查的對象字串”, “要搜查的關键句”); | string str = “I believe I can fly”; Console.WriteLine(Regex.IsMatch(“believe”)); //結果: True |
撷取字串: String.Substring(Int32, Int32); String.Substring(由第幾個字元開始撷取, 要撷取多少個); | string str = “I believe I can fly”; Console.WriteLine(str.Substring(2, 7)); //結果: believe |
字串對比 (包含大小寫對比): String.Equals(String); String.Equals(“要對比的字串”); | string str1 = “I believe I can fly”; string str2 = “i believe i can fly”;Console.WriteLine(str1.Equals(str2)); //結果: False |
字串對比 (不包含大小寫對比): String.Equals(String, StringComparison); String.Equals(“要對比的字串”, StringComparison.CurrentCultureIgnoreCase); | string str1 = “I believe I can fly”; string str2 = “i believe i can fly”; Console.WriteLine(str1.Equals(str2, StringComparison.CurrentCultureIgnoreCase)); //結果: True |
字串對比: String.CompareTo(String); String.CompareTo(“要對比的字串”); *回傳值: 0= 相同; -1 = 不相同 | string str1 = “I believe I can fly”; string str2 = “I sure I can fly”; Console.WriteLine(str1.CompareTo(str2)); //結果: 0 |
字串是不是空值(null)或空字串(“”): String.IsNullOrEmpty(String); String.IsNullOrEmpty(“要對比的字串”); | string str = ” “;Console.WriteLine(String.IsNullOrEmpty(str)); //結果: False |
字串是不是空值(null)或空字串(“”)或空格(” “) String.IsNullOrWhiteSpace(String); String.IsNullOrWhiteSpace(“要對比的字串”); | string str = ” “; Console.WriteLine(String.IsNullOrWhiteSpace(str)); //結果: True |
字串組合: String.Concat(String, String); String.Concat(“字串1”, “字串2”); | string str1 = “I believe I can fly. “; string str2 = “I believe I can talk to sky. “; Console.WriteLine(String.Concat(str1, str2)); //結果: “I believe I can fly. I believe I can talk to // sky.” |
字串數組組合: String.Join(String, String[]); String.Join(“接合字串”, “字串數組”); | string[] strs = new string[] { “I”, “believe”, “I”, “can”, “fly.” }; Console.WriteLine(String.Join(” “, strs)); //結果: “I believe I can fly. |
其他相關:
C# 字串String功能速查表 – 插入, 移除, 取代, 移除空白, 補上空白, 轉換成大小寫, 合併分割
參考資料:https://docs.microsoft.com/en-us/dotnet/api/system.string?view=net-6.0