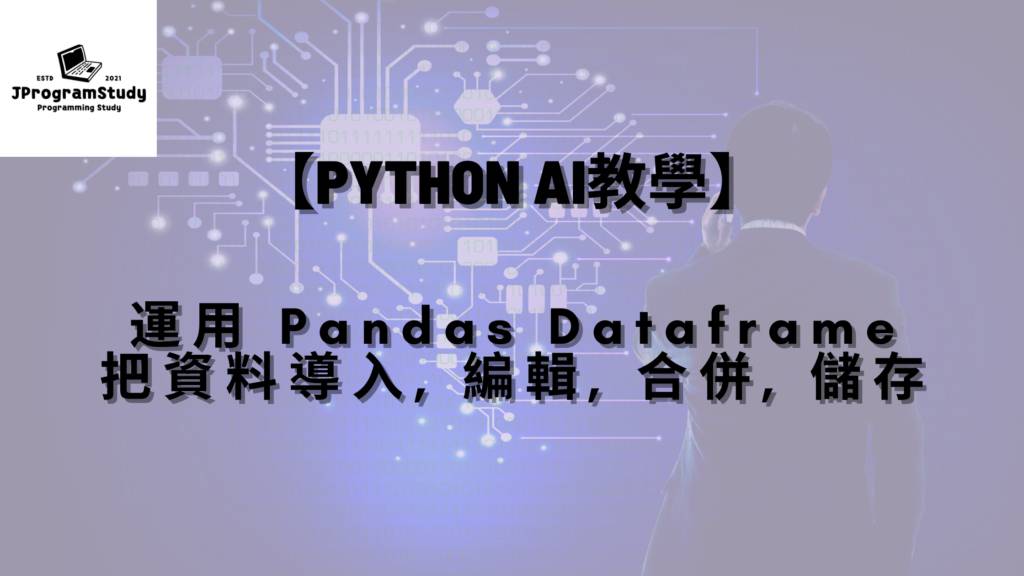
Pandas Dataframe – 在本文中,我將向你展示利用Python AI中Pandas資料庫的Dataframe, 來進行資料導入, 編輯, 合併, 及儲存。
安裝資料庫 | pip install pandas |
導入資料庫 | import pandas as pd |
把資料導入dataframe | |
設定Dictionary 資料 | students = { “name”: [“Johnson”, “Windy”, “Dickson”, “Jenny”], “age”: [14, 16, 14, 15], “email”: [“js@ptschool.com”, “wffy@gmail.com”, “dickson2004@gmail.com”, “hello12344321@hotmail.com”], “chinese”: [90, 89, 33, 78], “english”: [88, 56, 65, 67],} |
把Dictionary 資料導入dataframe | student_df = pd.DataFrame(students) |
資料編輯 | |
插入資料列 | new_df = df.append({ “name”: “Derekson”, “age”: 14, “email”: “d_chan@simpleABC.com”, “chinese”: 88, “english”: 97}, ignore_index=True) |
於第三欄後插入資料欄 | new_df.insert(3, column=”history”, value=[87, 44, 56, 66, 72]) |
刪除資料欄 (一欄) | new_df2 = new_df.drop([“history”], axis=1) |
刪除資料欄 (多欄) | new_df2 = new_df2 .drop([1, 2], axis=1) |
刪除資料列 (一列) | new_df2 = new_df.drop([0], axis=0) |
刪除資料列 (多列) | new_df2 = new_df2 .drop([1, 2], axis=0) |
刪除包含NaN或空值的資料 | new_df = new_df.dropna() |
刪除重複的資料 | new_df = new_df.drop_duplicates() |
把包含NaN或空值的資料設定為0 | new_df.fillna(0) |
找欄位出現過的值 (不重覆) | new_df.Sex.unique() |
重置DataFrame 的索引 | new_df.reset_index(inplace=True) |
依 DataFrame 欄位的內容分成分成兩個新個欄位 | new_df[[‘login_name’, ‘domain’]] = new_df.email.str.split(‘@’, expand=True) |
資料合併 | |
把dataframe 列位資料合併 | student_cc = pd.concat([student_df1 , df student_df2], ignore_index=True) |
重置DataFrame 的索引 | student_cc.reset_index(inplace=True) |
用SQL的方式把dataframe 的欄位資料合併 (merge) | student_mg = pd.merge( student_df_1, student_df_2, how=” inner “, on=”name”, indicator=True) #left:left outer join #right:right outer join #outer: full outer join #inner: inner join |
顯示出Dataframe欄位的資訊 | print(student_df.info()) |
顯示出Dataframe的統計資訊 | print(student_df.describe()) |
顯示出Dataframe的列數與欄數 | print(select_df.shape) |
顯示出Dataframe欄位的名稱 | print(student_df.columns) |
儲存 | |
將 DataFrame 儲存成 CSV 檔案 | student_df.to_csv(‘student_info.csv’) |